C++ Programming Language
Introduction
C++ is a powerful, general-purpose programming language that supports both procedural and object-oriented programming paradigms. It is an extension of the C programming language and is widely used for system programming, game development, and high-performance applications. This article will explore the key aspects, best practices, and provide code examples to illustrate the power of C++.
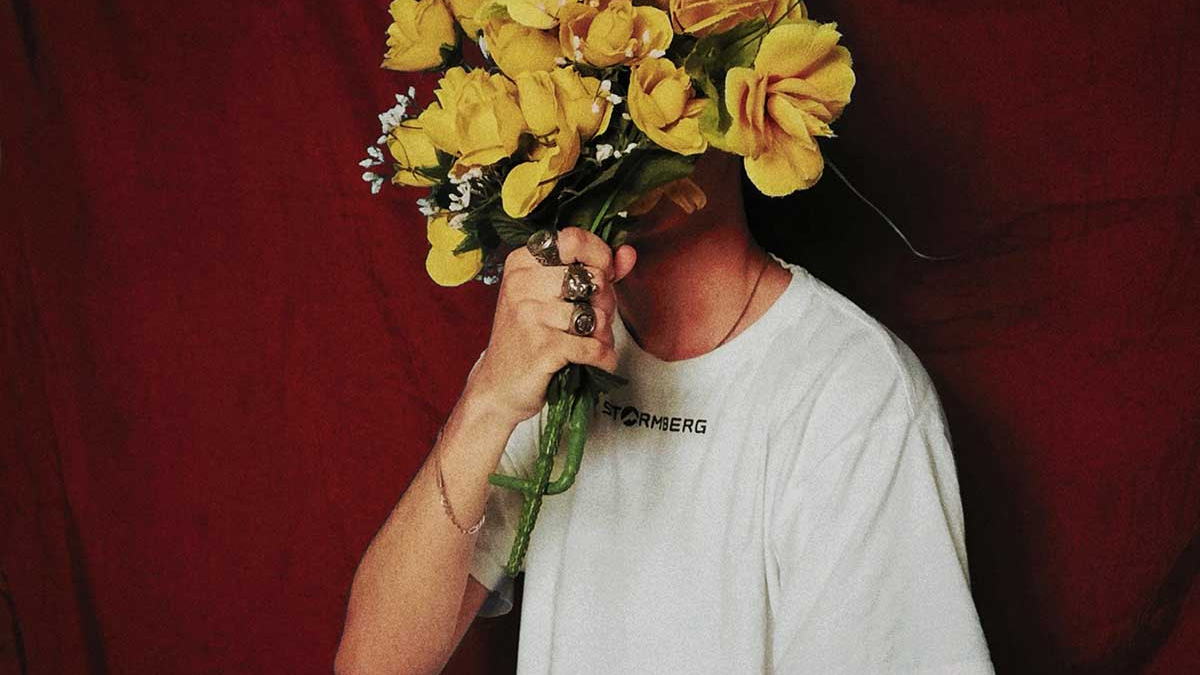
Basics
C++ is known for its efficiency and flexibility. It is a statically typed language, meaning that variable types are known at compile time. C++ supports a wide range of data types, including integers, floating-point numbers, characters, and booleans.
Key Aspects
- Data Types
- Variables and Constants
- Operators
Code Example
Control Structures
Control structures in C++ allow you to control the flow of your program. These include conditional statements (if, else, switch) and loops (for, while, do-while).
Key Aspects
- Conditional Statements
- Loops
Code Example
Functions
Functions in C++ allow you to encapsulate a block of code that can be reused throughout your program. Functions can take parameters and return values.
Key Aspects
- Function Declaration and Definition
- Function Overloading
- Inline Functions
Code Example
Pointers and References
Pointers and references are powerful features in C++ that allow you to manipulate memory directly. Pointers store the address of a variable, while references provide an alias for a variable.
Key Aspects
- Pointers
- References
- Dynamic Memory Allocation
Code Example
Classes and Objects
Classes and objects are the building blocks of object-oriented programming in C++. A class is a blueprint for creating objects, while an object is an instance of a class.
Key Aspects
- Class Definition
- Constructors and Destructors
- Member Functions
Code Example
Templates
Templates in C++ allow you to write generic code that can work with different data types. This is particularly useful for creating reusable functions and classes.
Key Aspects
- Function Templates
- Class Templates
Code Example
Best Practices
Following best practices is crucial for writing clean, maintainable, and efficient C++ code. Here are some best practices for C++ development:
C++ Best Practices
- Use meaningful variable and function names
- Follow the C++ Core Guidelines
- Write tests using frameworks like Google Test
- Use RAII (Resource Acquisition Is Initialization) for resource management
- Avoid raw pointers and use smart pointers (e.g., std::unique_ptr, std::shared_ptr)
Code Examples
Here are some code examples to illustrate the concepts discussed in this article.